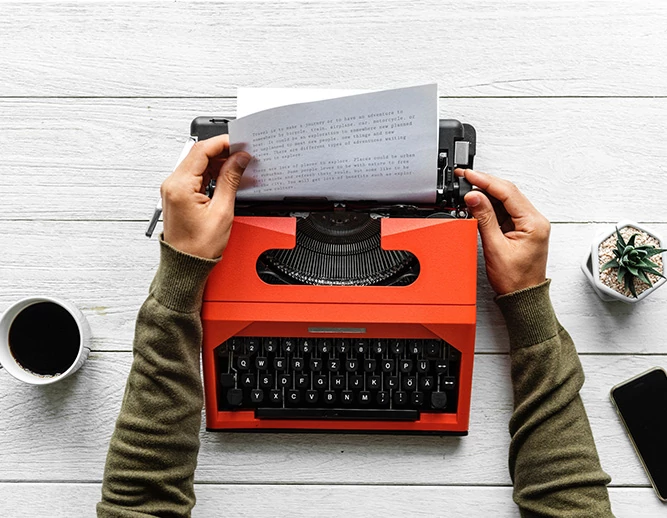
Caesar cipher encryption in Javascript
The caesar cipher is an encryption technique where we shift every letter in a given string by a given number for example the word 'Car' would become 'Dbs' if we shifted the letter by 1 and if we test the word 'Zoo' it become 'App' notice that the letter Z shifted to A.
Our code will handle negative numbers passed in and its a case-sensitive function.
Input : String , Integer number
Output : encrypted string.
Test Cases:
ceasarCipher("Zoo keeper",2);
// output : Bqq mggrgt
ceasarCipher("Javascript",-1);
// output: Izuzrbqhos
Implementation #
function ceasarCipher(str, num) {
// for passing negative and large values
num = num % 26;
var lowerCaseString = str.toLowerCase();
var alphabet = "abcdefghijklmnopqrstuvwxyz".split("");
var result = "";
for (var i = 0; i < lowerCaseString.length; i++) {
var currentLetter = lowerCaseString[i];
if (currentLetter === " ") {
result += currentLetter;
continue;
}
var currentIndex = alphabet.indexOf(currentLetter);
var shiftedIndex = currentIndex + num;
if (shiftedIndex > 25) shiftedIndex = shiftedIndex - 26;
if (shiftedIndex < 0) shiftedIndex = 26 + shiftedIndex;
if (str[i] === str[i].toUpperCase()) {
result += alphabet[shiftedIndex].toUpperCase();
} else {
result += alphabet[shiftedIndex];
}
}
return result;
}
The code is availalbe here : https://repl.it/@ahmd1/Caesar-Cipher-iAlgorithim
Credits #
Photo by rawpixel