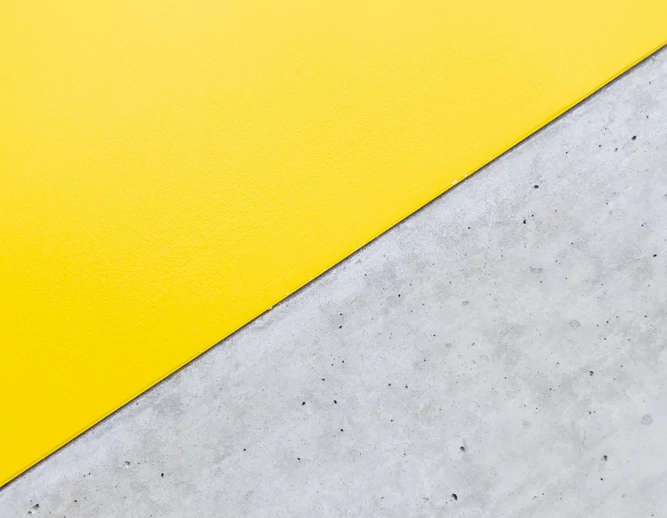
Mean Median Mode Algorithm
The Mean Median Mode Algorithm will take in a numeric array and return an object with Mean Median Mode of all the numbers in the array, you will learn some math calculation technique and functional programming, which means that you will break down your algorithm into seperate function so that these functions will be re-used multiple times.
Mean is the total sum of all numbers and divided by there count
Median is the middle value of the array and if the array size is even number will return the averge of both middel values
Mode will return the number which has the most occurence in the array
##Example
array: [1,2,3,4,5,6,4,1]
output: { mean: 3.25, median: 3.5, mode: [ '1', '4' ] }
Input : Array
Output : Object.
Implementation #
function meanMedianMode(array) {
return {
mean: getMean(array),
median: getMedian(array),
mode: getMode(array),
};
}
function getMean(array) {
var sum = 0;
array.forEach((element) => {
sum = element + sum;
});
var mean = sum / array.length;
return mean;
}
function getMedian(array) {
array.sort((a, b) => {
return a - b;
});
var median;
if (array.length % 2 != 0) {
median = array[Math.floor(array.length / 2)];
} else {
var firstMiddleValue = array[array.length / 2 - 1];
var secondMiddleValue = array[array.length / 2];
median = (firstMiddleValue + secondMiddleValue) / 2;
}
return median;
}
function getMode(array) {
var modeFrequencies = {};
array.forEach((element) => {
if (!modeFrequencies[element]) modeFrequencies[element] = 0;
modeFrequencies[element]++;
});
var maxFrequency = 0;
var modes = [];
for (var element in modeFrequencies) {
if (modeFrequencies[element] > maxFrequency) {
maxFrequency = modeFrequencies[element];
modes = [element];
} else if (modeFrequencies[element] == maxFrequency) {
modes.push(element);
}
// if all elements has the same frequency there will be no mode
if (modes.length == Object.keys(modeFrequencies).length) {
modes = [];
}
}
return modes;
}
Main Point: Math calculation technique and Functional Programming.
The code is availalbe here : https://repl.it/@ahmd1/Mean-Median-Mode
Credits #
Photo by Drew Beamer