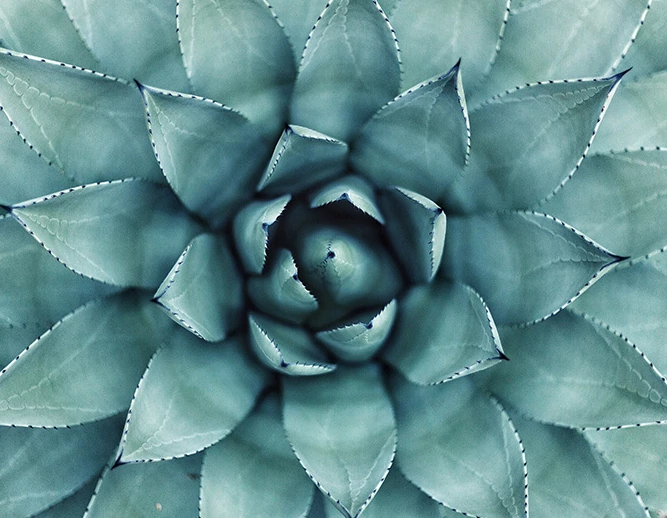
Check for palindromes with Javascript
A palindrome is a word, phrase, number, or other sequence of characters which reads the same backward or forward, For example the term race car is a palindrome because it spells out the same term whether it is written forward or if it is written backward.
Another example of a palindrome is the phrase Madam I'm Adam because it's the same both forwards and backwards.
Input : String
Output : return true if its a palindrome and fales if its not.
Implementation #
function isPalindrome(word) {
var charArray = word.toLowerCase().split("");
var validChar = "abcdefghijklmnopqrstuvwxyz";
var originalWord = "";
var reversedWord = "";
charArray.forEach((char) => {
if (validChar.indexOf(char) > -1) {
reversedWord = char + reversedWord;
originalWord = originalWord + char;
}
});
if (reversedWord == originalWord) return true;
else return false;
}
Main Point: Using array and string manipulation
The code is availalbe here : https://repl.it/@ahmd1/Palindrome
There are so many ways to check for palindromes for examples using regular expression so feel free to submit your solution with any programming language.
Credits #
Photo by Erol Ahmed